Visible to Intel only — GUID: GUID-E75496CB-3E31-4A8F-A34F-A55103B2375A
Visible to Intel only — GUID: GUID-E75496CB-3E31-4A8F-A34F-A55103B2375A
EigenValsVecs
Calculates eigen values and eigen vectors of image blocks for corner detection.
Syntax
IppStatus ippiEigenValsVecs_8u32f_C1R(const Ipp8u* pSrc, int srcStep, Ipp32f* pEigenVV, int eigStep, IppiSize roiSize, IppiKernelType kernType, int apertureSize, int avgWindow, Ipp8u* pBuffer);
IppStatus ippiEigenValsVecs_32f_C1R(const Ipp32f* pSrc, int srcStep, Ipp32f* pEigenVV, int eigStep, IppiSize roiSize, IppiKernelType kernType, int apertureSize, int avgWindow, Ipp8u* pBuffer);
Include Files
ippcv.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h, ippi.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib, ippi.lib
Parameters
- pSrc
- Pointer to the source image ROI.
- srcStep
- Distance, in bytes, between the starting points of consecutive lines in the source image.
- pEigenVV
- Image to store the results.
- eigStep
- Distance, in bytes, between the starting points of consecutive lines in the output image.
- roiSize
- Size of the source image ROI, in pixels.
- kernType
-
Specifies the type of kernel used to compute derivatives, possible values are:
ippKernelSobel
Sobel kernel 3x3 or 5x5 ippKernelSobelNeg
Negative Sobel kernel 3x3 or 5x5 ippKernelScharr
Scharr kernel 3x3 - apertureSize
- Size of the derivative operator in pixels, possible values are 3 or 5.
- avgWindow
- Size of the blurring window in pixels, possible values are 3 or 5.
- pBuffer
- Pointer to the temporary buffer.
Description
This function operates with ROI (see Regions of Interest in Intel IPP).
This function takes a block around the pixel and computes the first derivatives Dx and D y. This operation is performed for every pixel of the image using either Sobel or Scharr kernel in accordance with the kernType parameter. The apertureSize parameter specifies the size of the Sobel kernel. If this parameter is set to 3, the function used 3x3 kernel, if it is set to 5, the function uses 5x5 kernel. Only 3x3 size is available for the Scharr kernel, therefore the parameter apertureSize must be set to 3 if the Scharr kernel is used.
If the parameter apertureSize is set to 5 for operation with the Scharr kernel, the function returns error status.
The function computes eigen values and vectors of the following matrix:
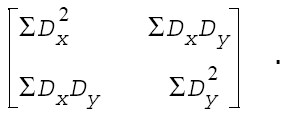
The summation is performed over the full block with averaging over the blurring window with size avgWindow.
The image eigenVV has the following format. For every pixel of the source image it contains six floating-point values - λ1, λ2 , x1, y1, x2, y2. These values are defined as follows:
λ1, λ2 |
Eigen values of the above matrix (λ1 ≥λ2 ≥ 0). |
x1, y1 |
Coordinates of the normalized eigen vector corresponding to λ1. |
x2, y2 |
Coordinates of the normalized eigen vector corresponding to λ2. |
In case of a singular matrix or when one eigen value is much smaller than the second one, all these six values are set to 0.
The function requires a temporary working buffer; its size should be computed previously by calling the function ippiEigenValsVecsGetBufferSize.
The parameters apertureSize and avgWindow must be the same for both functions ippiEigenValsVecsGetBufferSize and ippiEigenValsVecs.
Return Values
ippStsNoErr |
Indicates no error. Any other value indicates an error or a warning. |
ippStsNullPtrErr |
Indicates an error condition if one of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error condition if pRoiSize has a field with zero or negative value, or if apertureSize or avgWindow has an illegal value; or if kernType has wrong value. |
ippStsStepErr |
Indicates an error condition if srcStep is less than roiSize.width*<pixelSize>, or eigStep is less than roiSize.width*sizeof(Ipp32f)*6. |
ippStsNotEvenStepErr |
Indicates an error condition if steps for floating-point images are not divisible by 4. |
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
// A simple example of calculating eigen values and eigen vectors
// using Intel(R) Integrated Performance Primitives (Intel(R) IPP) functions:
// ippiEigenValsVecsGetBufferSize_8u32f_C1R
// ippiEigenValsVecs_8u32f_C1R
#include <stdio.h>
#include "ipp.h"
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) IPP function returned status different from ippStsNoErr */
/* Results of ippMalloc() are not validated because Intel(R) IPP functions perform bad arguments check and will return an appropriate status */
int main(void)
{
IppStatus status = ippStsNoErr;
Ipp8u pSrc[4 * 3] = /* Pointers to source images */
{ 2, 17, 2, 21,
9, 4, 11, 5,
2, 32, 7, 2 };
Ipp32f pEigenVV[24 * 3]; /* Image of eigen values and eigen vectors */
IppiSize roiSize = { 4, 3 };/* Size of source ROI in pixels */
int apertureSize = 3; /* Linear size of derivative filter aperture */
int avgWindow = 3; /* Linear size of averaging window */
int pBufferSize = 0; /* Pointer to work buffer */
Ipp8u* pBuffer = NULL;
/* Calculate size of temporary buffer */
check_sts( status = ippiEigenValsVecsGetBufferSize_8u32f_C1R(roiSize, apertureSize, avgWindow, &pBufferSize) )
pBuffer = ippsMalloc_8u(pBufferSize);
/* Calculate both eigen values and eigen vectors of 2x2 autocorrelation */
/* Gradient matrix for every pixel. */
check_sts( status = ippiEigenValsVecs_8u32f_C1R(pSrc, 4, pEigenVV, 24 * sizeof(Ipp32f), roiSize, ippKernelSobel, apertureSize, avgWindow, pBuffer) )
EXIT_MAIN
ippsFree(pBuffer);
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return (int)status;
}