Visible to Intel only — GUID: GUID-596E90C5-7944-48B9-AC8F-CB1039F64EA9
Visible to Intel only — GUID: GUID-596E90C5-7944-48B9-AC8F-CB1039F64EA9
FastN
Detects corners in an image using the FastN algorithm.
Syntax
IppStatus ippiFastN_8u_C1R(const Ipp8u* pSrc, int srcStep, Ipp8u* pDstCorner, int dstCornerStep, Ipp8u* pDstScore, int dstScoreStep, int* pNumCorner, IppiPoint srcRoiOffset, IppiSize dstRoiSize, IppiFastNSpec* pSpec, Ipp8u* pBuffer);
Include Files
ippcv.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h, ippi.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib, ippi.lib
Parameters
- pSrc
-
Pointer to the source image.
- srcStep
-
Distance, in bytes, between the starting points of consecutive lines in the source image.
- pDstCorner
-
Pointer to the destination image with corners.
- dstCornerStep
-
Distance, in bytes, between the starting points of consecutive lines in the destination image with corners.
- pDstScore
-
Pointer to the destination image with scores.
- dstScoreStep
-
Distance, in bytes, between the starting points of consecutive lines in the destination image with scores.
- pNumCorner
-
Pointer to the calculated number of corners.
- srcRoiOffset
-
Offset in the source image.
- dstRoiSize
-
Size of the destination ROI, in pixels.
- pSpec
-
Pointer to the specification structure.
- pBuffer
-
Pointer to the work buffer.
Description
The ippiFastN function implements the FastN corner detection algorithm. This function detects corners in the source image, calculates orientation and score of corners.
The figures below show pixels location for different radius values.
Radius = 1
Radius = 2

Radius = 3

Radius = 5

Radius = 7

Radius = 9
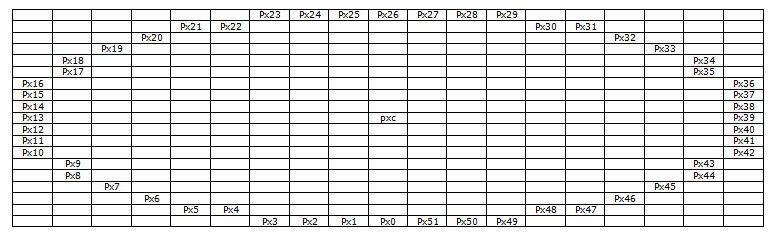
The function defines a pixel as a corner if the value of N consecutive pixels located on the circle with the center at the current pixel is greater (less) than the value of the current - central pixel. Differences between the values of pixels on the circle and the central pixel value must be greater than the threshold value. In this case, the corresponding pixel of the pDstCorner image is set to the following values in binary format:
If greater - 01xxxxxx
If less - 10xxxxxx
If the IPP_FASTN_ORIENTATION mode is not set, xxxxxx = 000000, otherwise, xxxxxx = the number of sector (bin) to which the corner directs. The orientationBin parameter defines the number of sectors that is computed from the bottom clockwise. If the source pixel is not a corner, the corresponding pixel of pDstCorner is set to zero.
If the IPP_FASTN_SCORE_MODE0 mode is set, the corresponding pixel of pDstCorner is set to the corner score. Score is a maximum among minimal differences with threshold calculated for every N consecutive pixels. If the source pixel is not a corner, the corresponding pixel of pDstScore is set to zero.
If the IPP_FASTN_NMS mode is set, the corners that have a corner with the greater score among neighboring pixels are cancelled.
Return Values
- ippStsNoErr
- Indicates no error. Any other value indicates an error.
- ippStsNullPtrErr
-
Indicates an error when:
pSrc, pDstCorner, pNumCorner, pSpec, or pBuffer is NULL
pDstScore is NULL if option is not equal to IPP_FASTN_SCORE_MODE0
- ippStsSizeErr
- Indicates an error when dstRoiSize is less than, or equal to zero.
- ippDataTypeErr
- Indicates an error when dataType has an illegal value.
- ippNumChannelsErr
- Indicates an error when numChannels has an illegal value.
- ippOutOfRangeErr
- Indicates an error when orientationBins or N has an illegal value.
- ippBadArgsErr
- Indicates an error when option or circleRadius has an illegal value.
- ippThresholdErr
- Indicates an error when threshold is negative.
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
// A simple example of the corner finding FastN algorithm using Intel(R) Integrated Performance Primitives (Intel(R) IPP) functions:
// ippiFastNGetSize
// ippiFastNInit
// ippiFastNGetBufferSize
// ippiFastN_8u_C1R
// ippiFastN2DToVec_8u
#include <stdio.h>
#include "ipp.h"
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) IPP function returned status different from ippStsNoErr */
/* Results of ippMalloc() are not validated because Intel(R) IPP functions perform bad arguments check and will return an appropriate status */
Ipp8u pSrc[16 * 16] = {/* Pointer to source images */
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 70, 70, 70, 70, 70, 70,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255,
255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255,
255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255, 255,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 255, 100, 100, 100, 100, 100,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 100, 100, 100, 100, 100, 100,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 100, 100, 100, 100, 100, 100,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 100, 100, 100, 100, 100, 100,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 100, 100, 100, 100, 100, 100,
10, 10, 10, 10, 10, 10, 255, 255, 255, 255, 100, 100, 100, 100, 100, 100
};
IppiSize srcSize = { 16 , 16 }; /* Size of source/destination ROI in pixels */
IppiSize dstSize = { 16, 16 };
IppStatus status = ippStsNoErr;
Ipp8u pDstCorner[16 * 16]; /* Pointer to destination images */
IppiCornerFastN *pDstStruct = NULL;
int srcStep = 16, dstCornerStep = 16; /* Steps, in bytes, through the source/destination images */
int circleRadius = 3; /* Radius for corner finding */
int N = 9; /* Critical number of pixels that have different value */
int orientationBins = 64; /* Number bins for defining direction */
int option = IPP_FASTN_CIRCLE; /* Defines the mode of processing */
const IppDataType dataType = ipp8u;
int nChannels = 1;
int specSize = 0; /* Size, in bytes, of the spec */
IppiFastNSpec *pSpec = NULL; /* Pointer to Spec */
Ipp32f threshold = 50.0f; /* Level for definition of critical pixels */
int bufSize = 0; /* Work buffer size */
Ipp8u *pBuffer = NULL; /* Pointer to the work buffer */
int maxLen = 0; /* Length of array of structures */
IppiPoint srcRoiOffset = { 0, 0 }; /* Offset in source image */
int numRealCorners; /* Number of corners */
int main(void)
{
/* Initialization of Spec for FastN */
check_sts( status = ippiFastNGetSize(srcSize, circleRadius, N, orientationBins, option, dataType, nChannels, &specSize) )
pSpec = (IppiFastNSpec *)ippsMalloc_8u(specSize);
/* Define spec size for FastN */
check_sts( status = ippiFastNInit(srcSize, circleRadius, N, orientationBins, option, threshold, dataType, nChannels, pSpec) )
check_sts( status = ippiFastNGetBufferSize(pSpec, dstSize, &bufSize) )
pBuffer = ippsMalloc_8u(bufSize);
/* Define size of working buffer for FastN */
check_sts( status = ippiFastN_8u_C1R(pSrc, srcStep, pDstCorner, dstCornerStep, NULL, 0, &maxLen, srcRoiOffset, dstSize, pSpec, pBuffer) )
pDstStruct = (IppiCornerFastN *)ippsMalloc_8u(sizeof(IppiCornerFastN)*maxLen);
/* Corner finding with FastN */
check_sts( status = ippiFastN2DToVec_8u(pDstCorner, dstCornerStep, NULL, 0, pDstStruct, dstSize, maxLen, &numRealCorners, pSpec) )
EXIT_MAIN
ippsFree(pDstStruct);
ippsFree(pBuffer);
ippsFree(pSpec);
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return (int)status;
}