Visible to Intel only — GUID: GUID-13E93729-3AF6-43A3-9147-4569B965BC15
Visible to Intel only — GUID: GUID-13E93729-3AF6-43A3-9147-4569B965BC15
MorphReconstructDilate
Reconstructs an image by dilation.
Syntax
IppStatus ippiMorphReconstructDilate_<mod>(const Ipp<datatype>* pSrc, int srcStep, Ipp<datatype>* pSrcDst, int srcDstStep, IppiSize roiSize, Ipp8u* pBuffer, IppiNorm norm);
Supported values for mod:
8u_C1IR | 16u_C1IR | 64f_C1IR |
IppStatus ippiMorphReconstructDilate_32f_C1IR(const Ipp32f* pSrc, int srcStep, Ipp32f* pSrcDst, int srcDstStep, IppiSize roiSize, Ipp32f* pBuffer, IppiNorm norm);
Include Files
ippcv.h
Domain Dependencies
Headers: ippcore.h, ippvm.h, ipps.h, ippi.h
Libraries: ippcore.lib, ippvm.lib, ipps.lib, ippi.lib
Parameters
pSrc |
Pointer to the source image ROI. |
||||
srcStep |
Distance in bytes between starts of consecutive lines in the source image. |
||||
pSrcDst |
Pointer to the descreased and reconstructed image ROI. |
||||
srcDstStep |
Distance in bytes between starts of consecutive lines in the decreased and reconstructed image. |
||||
roiSize |
Size of the source and destination image ROI. |
||||
norm |
Type of norm to form the mask for dilation; the following values are possible:
|
||||
pBuffer |
Pointer to the buffer. |
Description
This function operates with ROI (see Regions of Interest in Intel IPP).
This function performs morphological reconstruction of the decreased source image by dilation [Vincent93]. The operation is performed in the working buffer whose size should be computed using the function MorphReconstructGetBufferSize beforehand.
This operation enables detection of the regional maximums that can be used as markers for successive watershed segmentation.
Example below shows how the morphological reconstruction can be used to build markers of objects with different brightness. Some value (cap size) is subtracted from the initial image and then the subtracted image is reconstructed to the initial one. Thresholding and opening complete the building of markers. The figure below shows the results of these operations.
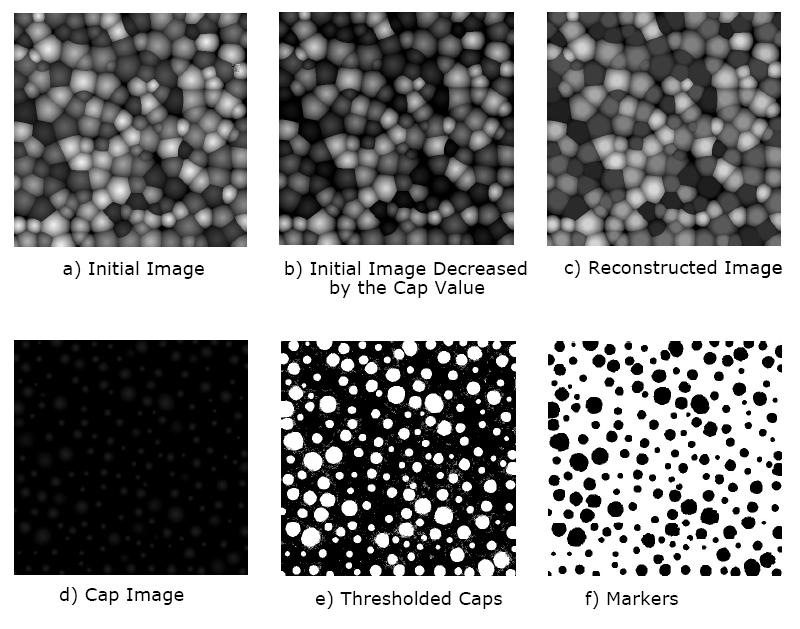
Return Values
ippStsNoErr |
Indicates no error. Any other value indicates an error or a warning. |
ippStsNullPtrErr |
Indicates an error condition if one of the specified pointers is NULL. |
ippStsSizeErr |
Indicates an error condition if roiSize has a field with a zero or negative value. |
ippStsStepErr |
Indicates an error condition if srcStep or srcDstStep is less than roiSize.width * <pixelSize>. |
ippStsNotEvenStepErr |
Indicates an error condition if one of the step values is not divisible by 4 for floating-point images. |
ippStsBadArgErr |
Indicates an error condition if norm has an illegal value. |
Example
/*******************************************************************************
* Copyright 2015 Intel Corporation.
*
*
* This software and the related documents are Intel copyrighted materials, and your use of them is governed by
* the express license under which they were provided to you ('License'). Unless the License provides otherwise,
* you may not use, modify, copy, publish, distribute, disclose or transmit this software or the related
* documents without Intel's prior written permission.
* This software and the related documents are provided as is, with no express or implied warranties, other than
* those that are expressly stated in the License.
*******************************************************************************/
// Performs morphological reconstruction of pSrcDst under/above pSrc
// ippiMorphReconstructGetBufferSize
// ippiMorphReconstructDilate_8u_C1IR
#include <stdio.h>
#include "ipp.h"
#define WIDTH 128 /* Image width */
#define HEIGHT 128 /* Image height */
#define KERSIZE 3 /* Kernel size */
/* Next two defines are created to simplify code reading and understanding */
#define EXIT_MAIN exitLine: /* Label for Exit */
#define check_sts(st) if((st) != ippStsNoErr) goto exitLine; /* Go to Exit if Intel(R) Integrated Performance Primitives (Intel(R) IPP) function returned status different from ippStsNoErr */
/* Results of ippMalloc() are not validated because Intel(R) IPP functions perform bad arguments check and will return an appropriate status */
int main(void)
{
IppStatus status = ippStsNoErr;
IppiMorphAdvState *pState = NULL;
IppiSize roiSize= { WIDTH, HEIGHT }; /* image size */
IppiSize maskSize={3,3}; /* mask size */
Ipp8u *pSrc = NULL, *pDst = NULL, *pImg = NULL; /* initial and working image */
Ipp8u *pBuf = NULL, *pAdvBuf = NULL, pMask[9]={1,1,1,1,1,1,1,1,1};
int srcStep = 0; /* srcStep, in bytes, through the source image */
int size = 0, specSize = 0, bufferSize = 0; /* working buffers size */
int cap = 100; /* cap value */
pSrc = ippiMalloc_8u_C1(roiSize.width, roiSize.height, &srcStep);
pDst = ippiMalloc_8u_C1(roiSize.width, roiSize.height, &srcStep);
pImg = ippiMalloc_8u_C1(roiSize.width, roiSize.height, &srcStep);
check_sts( status = ippiMorphReconstructGetBufferSize(roiSize, ipp8u, 1, &size) )
pBuf = ippsMalloc_8u(size);
check_sts( status = ippiMorphAdvGetSize_8u_C1R( roiSize, maskSize, &specSize, &bufferSize ) )
pState = (IppiMorphAdvState*)ippsMalloc_8u(specSize);
pAdvBuf = (Ipp8u*)ippsMalloc_8u(bufferSize);
check_sts( status = ippiMorphAdvInit_8u_C1R( roiSize, pMask, maskSize, pState, pAdvBuf ) )
check_sts( status = ippiCopy_8u_C1R(pSrc, srcStep, pDst, srcStep, roiSize) )
check_sts( status = ippiCopy_8u_C1R(pSrc, srcStep, pImg, srcStep, roiSize) )
/* subtract cap size */
check_sts( status = ippiSubC_8u_C1IRSfs(cap, pDst, srcStep, roiSize, 0) )
/* reconstruct image */
check_sts( status = ippiMorphReconstructDilate_8u_C1IR(pSrc, srcStep, pDst, srcStep, roiSize, pBuf, (IppiNorm)ippiNormL1) )
/* get caps */
check_sts( status = ippiSub_8u_C1IRSfs(pDst, srcStep, pImg, srcStep, roiSize, 0) )
/* caps to white */
check_sts( status = ippiThreshold_GTVal_8u_C1IR(pImg, srcStep, roiSize, 0, 255) )
/* delete noise */
check_sts( status = ippiMorphOpenBorder_8u_C1R(pImg, srcStep, pDst, srcStep, roiSize,
ippBorderRepl, 0, pState, pAdvBuf) )
/* revert to get markers */
check_sts( status = ippiXorC_8u_C1IR(0xff, pDst, srcStep, roiSize) )
EXIT_MAIN
ippsFree(pBuf);
ippsFree(pState);
ippsFree(pAdvBuf);
ippiFree(pSrc);
ippiFree(pDst);
ippiFree(pImg);
printf("Exit status %d (%s)\n", (int)status, ippGetStatusString(status));
return status;
}